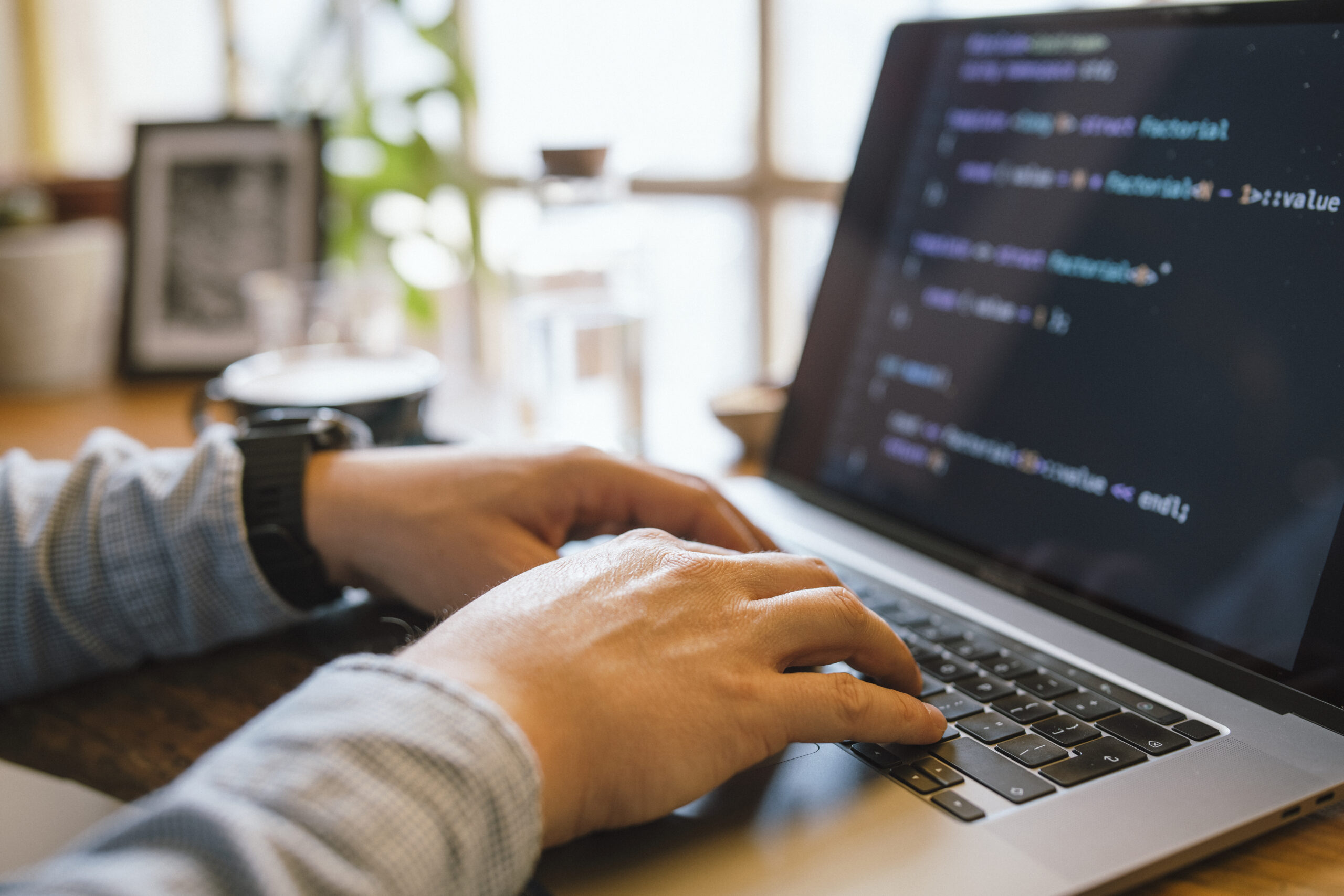
Debugging is one of the most vital — nonetheless frequently disregarded — capabilities in a very developer’s toolkit. It's not just about fixing broken code; it’s about comprehending how and why items go Mistaken, and Mastering to Assume methodically to unravel challenges successfully. Irrespective of whether you are a starter or maybe a seasoned developer, sharpening your debugging competencies can help you save several hours of annoyance and considerably transform your productiveness. Allow me to share many approaches to help you developers level up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
One of several fastest techniques developers can elevate their debugging skills is by mastering the applications they use on a daily basis. Though producing code is one particular Portion of advancement, understanding ways to communicate with it properly for the duration of execution is equally essential. Contemporary development environments occur Geared up with highly effective debugging capabilities — but many builders only scratch the surface area of what these tools can perform.
Just take, for instance, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment allow you to established breakpoints, inspect the value of variables at runtime, phase via code line by line, and even modify code over the fly. When utilized correctly, they let you notice just how your code behaves in the course of execution, which is priceless for tracking down elusive bugs.
Browser developer applications, for example Chrome DevTools, are indispensable for front-finish developers. They assist you to inspect the DOM, keep an eye on network requests, watch true-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and community tabs can change frustrating UI troubles into workable duties.
For backend or technique-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management around working procedures and memory administration. Learning these equipment could possibly have a steeper learning curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be relaxed with Variation control methods like Git to grasp code heritage, obtain the exact moment bugs were introduced, and isolate problematic adjustments.
Eventually, mastering your instruments suggests likely further than default configurations and shortcuts — it’s about developing an intimate knowledge of your improvement surroundings to ensure when troubles occur, you’re not missing in the dead of night. The higher you already know your instruments, the greater time it is possible to commit fixing the actual difficulty as opposed to fumbling by means of the process.
Reproduce the issue
Probably the most crucial — and often missed — ways in productive debugging is reproducing the situation. Ahead of jumping into the code or making guesses, builders need to have to make a constant environment or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug gets a recreation of opportunity, normally bringing about wasted time and fragile code variations.
Step one in reproducing an issue is accumulating just as much context as you possibly can. Talk to inquiries like: What actions triggered The problem? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it will become to isolate the exact circumstances less than which the bug happens.
As you’ve collected more than enough data, try to recreate the situation in your local natural environment. This could indicate inputting exactly the same facts, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, think about composing automatic exams that replicate the sting conditions or state transitions included. These checks not just enable expose the problem but in addition reduce regressions in the future.
Often, The difficulty might be setting-unique — it might take place only on selected functioning methods, browsers, or beneath unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is usually instrumental in replicating such bugs.
Reproducing the trouble isn’t only a action — it’s a mentality. It requires patience, observation, as well as a methodical technique. But when you finally can continually recreate the bug, you might be already halfway to fixing it. Having a reproducible scenario, You can utilize your debugging equipment far more properly, take a look at probable fixes safely and securely, and converse far more Plainly using your staff or buyers. It turns an summary criticism right into a concrete obstacle — Which’s the place developers thrive.
Read and Understand the Mistake Messages
Mistake messages in many cases are the most worthy clues a developer has when anything goes Mistaken. As an alternative to viewing them as irritating interruptions, developers should really study to deal with error messages as immediate communications with the technique. They usually tell you exactly what transpired, the place it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Begin by reading the information very carefully and in whole. A lot of developers, specially when below time pressure, look at the very first line and immediately start out producing assumptions. But deeper while in the error stack or logs may well lie the correct root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and understand them initially.
Break the mistake down into parts. Could it be a syntax error, a runtime exception, or possibly a logic error? Does it issue to a particular file and line selection? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also handy to know the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and learning to recognize these can drastically accelerate your debugging system.
Some mistakes are obscure or generic, As well as in those circumstances, it’s important to examine the context during which the mistake happened. Check connected log entries, enter values, and up to date modifications inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede greater difficulties and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Just about the most strong instruments in a very developer’s debugging toolkit. When applied correctly, it offers serious-time insights into how an software behaves, encouraging you have an understanding of what’s going on underneath the hood without having to pause execution or move in the code line by line.
A very good logging tactic commences with figuring out what to log and at what stage. Widespread logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts for the duration of growth, Data for common occasions (like successful get started-ups), Alert for prospective concerns that don’t break the applying, ERROR for real problems, and Lethal if the program can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure important messages and decelerate your program. Focus on important situations, condition modifications, enter/output values, and demanding decision details within your code.
Structure your log messages Obviously and regularly. Include things like context, for example timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
Throughout debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re In particular beneficial in generation environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, clever logging is about equilibrium and clarity. Having a very well-thought-out logging strategy, you could reduce the time it requires to identify issues, achieve further visibility into your applications, and improve the Total maintainability and trustworthiness of your code.
Feel Similar to a Detective
Debugging is not merely a technical activity—it is a method of investigation. To effectively recognize and correct bugs, builders must method the method just like a detective fixing a thriller. This mentality helps break down sophisticated troubles into workable sections and abide by clues logically to uncover the foundation cause.
Begin by gathering evidence. Consider the indicators of the situation: mistake messages, incorrect output, or effectiveness challenges. Identical to a detective surveys against the law scene, obtain just as much applicable information and facts as you can without jumping to conclusions. Use logs, test cases, and person experiences to piece alongside one another a clear photograph of what’s going on.
Future, sort hypotheses. Check with on your own: What may very well be triggering this conduct? Have any adjustments lately been produced to the codebase? Has this difficulty happened ahead of beneath comparable circumstances? The intention will be to slim down prospects and identify opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the issue in a managed surroundings. In the event you suspect a selected operate or component, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results direct you nearer to the truth.
Pay near interest to compact specifics. Bugs often disguise from the least envisioned areas—similar to a missing semicolon, an off-by-a person error, or a race issue. Be thorough and client, resisting the urge to patch the issue devoid of totally being familiar with it. Short term fixes may perhaps conceal the actual issue, just for it to resurface later.
And finally, keep notes on Whatever you tried using and realized. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future concerns and assistance Other people fully grasp your reasoning.
By thinking like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden troubles in elaborate methods.
Compose Assessments
Crafting tests is one of the best strategies to help your debugging skills and All round growth effectiveness. Checks don't just help catch bugs early but additionally serve as a safety Web that offers you confidence when creating modifications in your codebase. A properly-examined software is simpler to debug as it lets you click here pinpoint particularly wherever and when a dilemma takes place.
Get started with device assessments, which target specific features or modules. These modest, isolated assessments can swiftly reveal regardless of whether a particular piece of logic is working as expected. Any time a exam fails, you promptly know wherever to seem, drastically lowering time spent debugging. Device assessments are Specially beneficial for catching regression bugs—problems that reappear following Beforehand staying mounted.
Up coming, integrate integration checks and conclude-to-stop tests into your workflow. These assistance be sure that different elements of your software operate with each other smoothly. They’re specially beneficial for catching bugs that happen in elaborate programs with numerous factors or companies interacting. If some thing breaks, your checks can let you know which Element of the pipeline failed and under what ailments.
Creating checks also forces you to Assume critically about your code. To check a function thoroughly, you may need to understand its inputs, predicted outputs, and edge cases. This standard of comprehending Obviously prospects to higher code composition and less bugs.
When debugging a concern, writing a failing examination that reproduces the bug can be a strong starting point. Once the examination fails continuously, you are able to center on fixing the bug and observe your take a look at go when the issue is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In short, creating assessments turns debugging from the frustrating guessing recreation right into a structured and predictable system—helping you catch far more bugs, speedier and more reliably.
Consider Breaks
When debugging a difficult situation, it’s uncomplicated to be immersed in the problem—looking at your display for several hours, trying solution right after Resolution. But Among the most underrated debugging applications is solely stepping absent. Having breaks allows you reset your mind, reduce aggravation, and often see the issue from a new perspective.
If you're too close to the code for too long, cognitive exhaustion sets in. You might start overlooking obvious faults or misreading code that you choose to wrote just several hours before. With this condition, your brain turns into much less effective at problem-resolving. A brief stroll, a coffee break, or simply switching to another undertaking for 10–15 minutes can refresh your focus. Lots of builders report obtaining the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also support stop burnout, especially all through extended debugging sessions. Sitting down before a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent lets you return with renewed Power in addition to a clearer frame of mind. You could suddenly detect a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a great general guideline would be to established a timer—debug actively for 45–sixty minutes, then take a 5–ten minute crack. Use that time to maneuver around, extend, or do something unrelated to code. It could feel counterintuitive, Specially under restricted deadlines, but it in fact leads to more quickly and more practical debugging Over time.
Briefly, taking breaks is just not an indication of weakness—it’s a wise system. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel vision That always blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Each individual Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or simply a deep architectural issue, each one can educate you anything precious if you make an effort to mirror and examine what went Erroneous.
Get started by inquiring yourself a couple of crucial queries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are caught before with improved tactics like unit testing, code evaluations, or logging? The answers usually reveal blind spots inside your workflow or knowing and enable you to Construct more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent habit. Continue to keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll begin to see styles—recurring troubles or frequent blunders—that you could proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your friends might be Specifically powerful. Whether it’s via a Slack message, a brief publish-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar situation boosts team performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as essential areas of your improvement journey. In spite of everything, a number of the most effective developers are not the ones who generate best code, but those who continually learn from their problems.
Eventually, Each and every bug you take care of adds a different layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Strengthening your debugging competencies will take time, exercise, and tolerance — nevertheless the payoff is big. It will make you a more productive, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.